The Best Way to Work with ORM in .NET: A Practical Guide for Developers
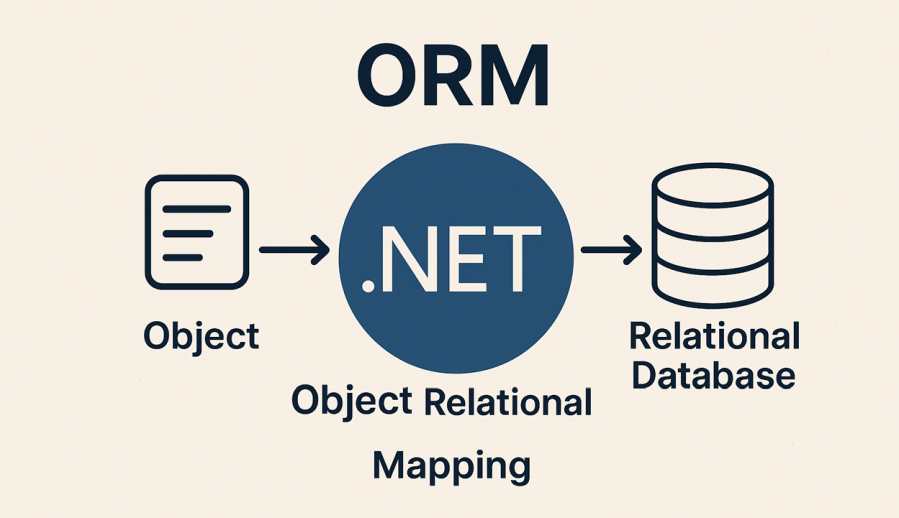
Ever wondered what ORM in .NET is and why developers rave about it? ORM, or Object-Relational Mapping, is a game-changer in modern software development, especially within the .NET Framework. It bridges the gap between the object model used in C# applications and the relational database that stores persistent data. Introduced to simplify data access layers, ORM removes the repetitive, error-prone SQL code by offering a higher-level abstraction.
Originally, developers had to manually write SQL to map objects to tables—time-consuming and tedious. ORM tools changed that by automating entity mapping, improving developer productivity, and enabling a cleaner, testable architecture using patterns like the repository pattern.
In .NET, ORM is vital in managing the data layer, allowing you to focus more on business logic than database plumbing. Whether you’re a beginner exploring the ORM basics or a pro refining your architecture, understanding how ORM works in .NET is essential.
This article offers a deep overview of ORM in C#, its purpose, history, and real-world benefits. Let’s break down the ORM meaning in .NET, and explore where it fits into your .NET projects.
Choosing the Right ORM for .NET Projects
When it comes to selecting the best ORM for .NET, there’s no one-size-fits-all answer—it depends on your project’s needs. Entity Framework Core (EF Core) is the go-to for most enterprise apps thanks to its rich features, ease of use, and strong integration with the .NET Framework. It’s perfect for developers seeking rapid development and maintainability with minimal SQL.
If raw speed and control matter, Dapper—a micro-ORM—is a top pick. It’s lightweight, blazing-fast, and great for performance-critical apps, but it requires more hands-on SQL and has a steeper learning curve for complex queries.
NHibernate, though more complex, shines in advanced scenarios needing fine-tuned mapping and legacy database support. It offers robust scalability and a mature ecosystem but comes with higher complexity and setup time.
LINQ to SQL is simple and works well for small to mid-sized projects, but its limited feature set and lack of ongoing support make it less ideal for larger applications.
When you compare ORMs for C#, consider the performance tradeoffs, app size, and long-term maintainability. Whether it’s Entity Framework vs Dapper or evaluating other ORM options in .NET, choose one that aligns with your architecture, team skill level, and project goals.
Visual Setting Up ORM in a .NET Project
Setting up an ORM in your .NET project doesn’t have to be intimidating—especially when using Entity Framework paired with a visual tool like Entity Developer. This combo makes it incredibly easy to bridge your object model with a relational database, even if you’re not a database expert. Let’s walk through a simplified, visual-first setup to get your ORM layer up and running in no time.
Install Entity Developer: Begin by installing Entity Developer—an intuitive visual designer for ORM models. It integrates seamlessly with Visual Studio and supports multiple ORM tools including Entity Framework.
Connect to Your Database via dotConnect: Open Entity Developer, create a new model and connect to your data database or other supported source. With just a few clicks, you can generate a model from the data source, automatically pulling tables, views, and relationships.
Visualize and Edit Your Model: Use the drag-and-drop interface to organize your classes. Edit properties, define complex types, or add enums visually. This speeds up development and avoids human error.
Flexible Mapping: Easily adjust how classes and properties map to your database tables and columns. You get full control over entity mapping—without diving into configuration files.
This visual setup boosts productivity, simplifies onboarding for new developers, and ensures a clean, maintainable data layer in your .NET app.
ORM Best Practices in .NET
Mastering ORM isn’t just about plugging in a library—it’s about using it smartly. For efficient ORM usage in .NET, a few best practices can make the difference between a sluggish app and a high-performance machine.
Start by avoiding lazy loading when possible. While convenient, it silently fires multiple queries, which can kill performance. Opt for eager loading using .Include() to pull related data in one go. Also, keep an eye on change tracking—disable it for read-only operations to reduce overhead.
Batching is your best friend. Instead of inserting or updating records one at a time, batch them. This simple tweak massively boosts ORM performance in C#. Use caching for frequently accessed data and ensure query optimization by analyzing execution plans or using database profiling tools.
Stick to clean architecture. Keep ORM logic out of your business layer. Follow patterns like repository and unit of work to maintain a modular structure. Avoid embedding validation logic in your data models—separate concerns cleanly.
Finally, steer clear of anti-patterns like overusing navigation properties or letting your entities grow bloated. These best ORM practices in .NET ensure your codebase is not just functional, but scalable and maintainable.
Future Trends in ORM and .NET
The ORM landscape in .NET is evolving fast, with exciting trends shaping the future of data access. With .NET 9 now in the spotlight, expect major performance improvements thanks to AOT (Ahead-of-Time) compilation and low-level performance APIs that significantly cut overhead for ORM operations.
One emerging shift is the rise of lightweight and hybrid ORMs, blending raw SQL power with high-level abstraction. These tools aim to combine the flexibility of Dapper with the structure of Entity Framework Core, giving developers more control without sacrificing maintainability.
Blazor is also pushing new demands on ORM tools—requiring client-friendly data patterns and efficient, asynchronous operations. Meanwhile, EF Core’s roadmap includes enhancements like better no-tracking query performance, advanced automation for schema migrations, and smarter caching strategies.
Open-source innovation is booming too. New ORM libraries and community-driven tools are rapidly iterating on what traditional ORMs can do, with a focus on modularity and cleaner architectures.
As .NET matures, the future of EF Core and ORM usage is geared toward high performance, developer experience, and tighter integration with modern development stacks. Keep an eye on these ORM trends in .NET—they’re set to redefine how we build data-driven applications.
Want Your Story Featured?
Get in front of thousands of founders, investors, PE firms, tech executives, decision makers, and tech readers by submitting your story to TechStartups.com.
Get Featured